สร้าง Fragment ขึ้นมาใหม่ชื่อ StaticFragment
ในไฟล์ activity_main.xml เรียกใช้ StaticFragment ซึ่งเป็นการเรียกใช้แบบ static
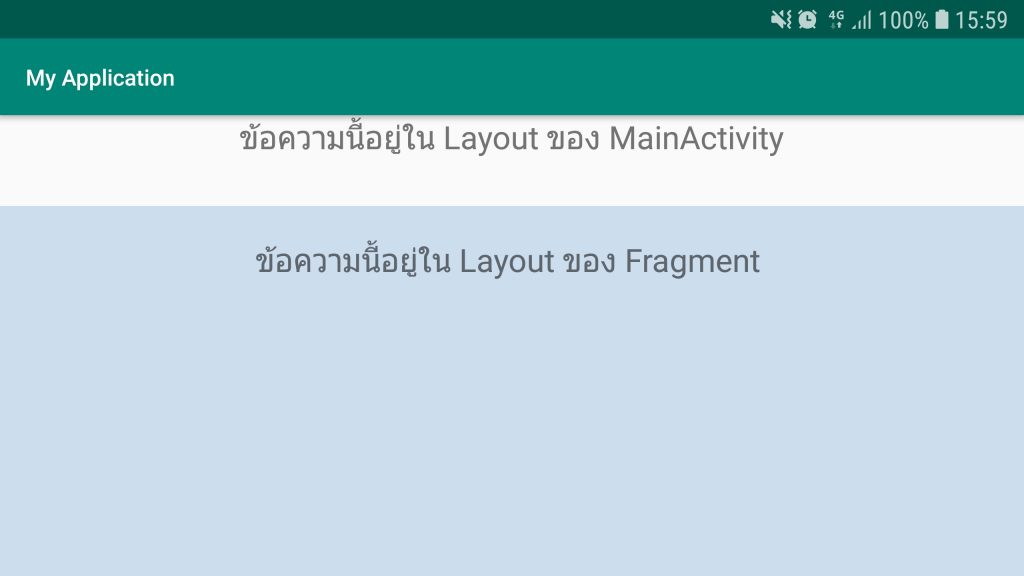
ไฟล์ที่เกี่ยวข้อง
- activity_main.xml
- MainActivity.java
- fragment_static.xml
- StaticFragment.java
สร้าง Fragment ชื่อ StaticFragment
New > Fragment > Fragment (Blank)

เอาเครื่องหมายถูกหน้า Include fragment factory methods? และ Include interface callback? ออก ไม่งั้นจะได้ generate code มาเยอะมาก
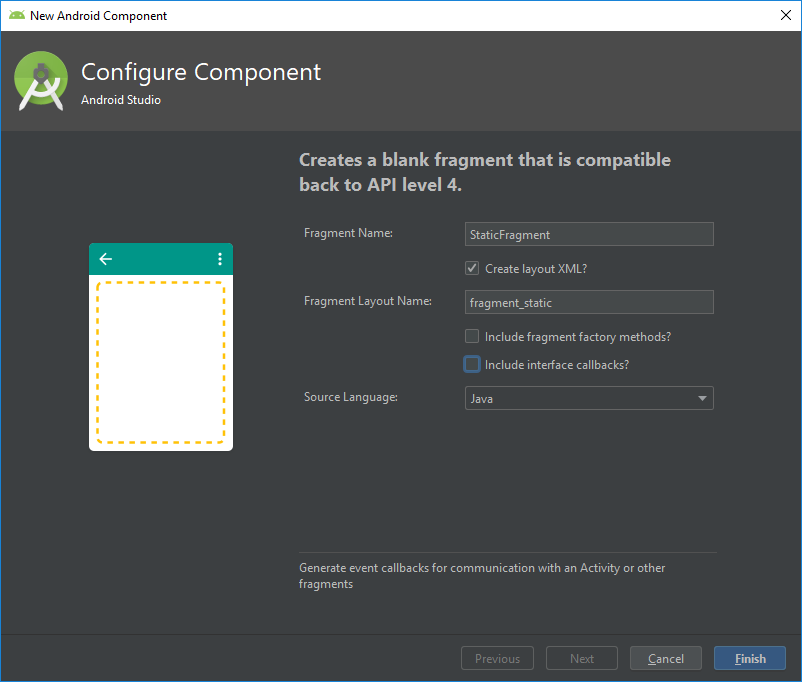
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_horizontal" android:layout_marginBottom="30dp" android:text="ข้อความนี้อยู่ใน Layout ของ MainActivity" android:textSize="20sp" /> <FrameLayout android:layout_width="match_parent" android:layout_height="match_parent"> <fragment android:id="@+id/fragmentMain" android:name="com.phaisarn.myapplication.StaticFragment" android:layout_width="match_parent" android:layout_height="match_parent" /> </FrameLayout> </LinearLayout> </androidx.constraintlayout.widget.ConstraintLayout>
บรรทัดที่ 26 กำหนด FrameLayout
ไว้ใส่ fragment
บรรทัดที่ 31 ไม่กำหนด id
ให้ fragment
แล้วจะ error ไม่ว่าจะเรียกใช้ fragment จาก code หรือไม่
บรรทัดที่ 32 เรียกใช้ fragment
ที่ต้องการ ซึ่งในที่นี้คือ com.phaisarn.myapplication.StaticFragment
MainActivity.java
package com.phaisarn.myapplication; import androidx.appcompat.app.AppCompatActivity; import androidx.fragment.app.Fragment; import android.os.Bundle; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Fragment fragment = getSupportFragmentManager().findFragmentById(R.id.fragmentMain); StaticFragment staticFragment = (StaticFragment) fragment; } }
บรรทัดที่ 15 การหา Fragment
ใช้ findFragmentById()
fragment_static.xml
<?xml version="1.0" encoding="utf-8"?> <FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#cde" tools:context=".StaticFragment"> <!-- TODO: Update blank fragment layout --> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_horizontal" android:layout_margin="20dp" android:text="ข้อความนี้อยู่ใน Layout ของ Fragment " android:textSize="20sp" /> </FrameLayout>
StaticFragment.java
package com.phaisarn.myapplication; import android.os.Bundle; import androidx.fragment.app.Fragment; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; /** * A simple {@link Fragment} subclass. */ public class StaticFragment extends Fragment { public StaticFragment() { // Required empty public constructor } @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { // Inflate the layout for this fragment View view = inflater.inflate(R.layout.fragment_static, container, false); return view; } }
หน้า Design
ถ้าดูที่หน้า design ส่วนที่เป็น fragment จะไม่ preview มีเพียงข้อความ fragment
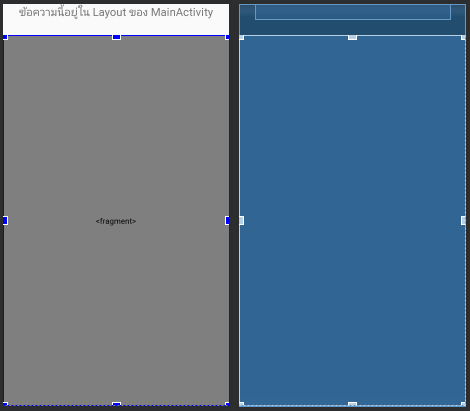
ถ้าจะให้ส่วนที่เป็น fragment ทำการ preview ให้กำหนดที่ tools:layout
<fragment android:id="@+id/fragmentMain" android:name="com.phaisarn.myapplication.StaticFragment" android:layout_width="match_parent" android:layout_height="match_parent" tools:layout="@layout/fragment_static" />

การอ้างถึง View ใน Fragment
เมื่อกดปุ่มให้แสดง Toast
ซึ่ง ปุ่มอยู่ใน Fragment

fragment_static.xml
<?xml version="1.0" encoding="utf-8"?> <FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#cde" tools:context=".StaticFragment"> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_horizontal" android:layout_margin="20dp" android:text="click Me!" android:textSize="20sp" /> </FrameLayout>
StaticFragment.java
package com.phaisarn.myapplication; import android.os.Bundle; import androidx.annotation.NonNull; import androidx.annotation.Nullable; import androidx.fragment.app.Fragment; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.Button; import android.widget.Toast; public class StaticFragment extends Fragment { public StaticFragment() { // Required empty public constructor } @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { View view = inflater.inflate(R.layout.fragment_static, container, false); return view; } @Override public void onViewCreated(@NonNull View view, @Nullable Bundle savedInstanceState) { super.onViewCreated(view, savedInstanceState); Button button1 = view.findViewById(R.id.button1); button1.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Toast.makeText(getContext(), "Hello World!", Toast.LENGTH_SHORT).show(); } }); } }