กดปุ่มแล้วจะแสดง dialog ถ้าตอบ Yes จะเปลี่ยนสี background ของ activity นี้เป็นสีแดง
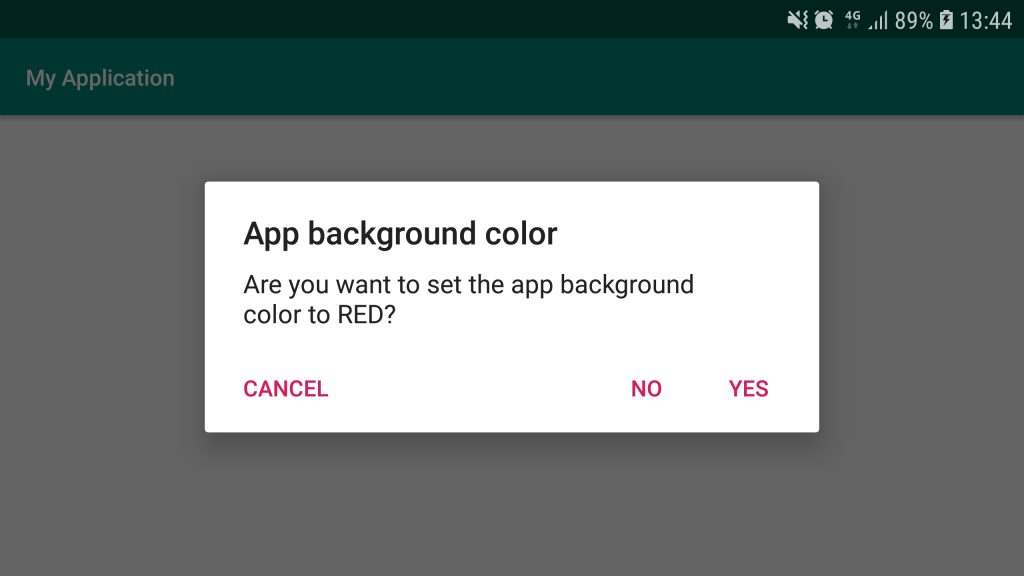
ไฟล์ที่เกี่ยวข้อง
- activity_main.xml
- MainActivity.kt
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" xmlns:app="http://schemas.android.com/apk/res-auto" android:id="@+id/layoutRoot" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <Button android:id="@+id/btnShowAlert" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Show Alert" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent"/> </androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.kt
package com.phaisarn.myapplication import android.graphics.Color import android.os.Bundle import android.widget.Toast import androidx.appcompat.app.AppCompatActivity import androidx.appcompat.app.AlertDialog import kotlinx.android.synthetic.main.activity_main.* class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) // when button is clicked, show the alert btnShowAlert.setOnClickListener { // Initialize a new instance of val builder = AlertDialog.Builder(this@MainActivity) // Set the alert dialog title builder.setTitle("App background color") // Display a message on alert dialog builder.setMessage("Are you want to set the app background color to RED?") // Set a positive button and its click listener on alert dialog builder.setPositiveButton("YES") { _, _ -> // Do something when user press the positive button Toast.makeText(applicationContext, "Ok, we change the app background.", Toast.LENGTH_SHORT).show() // Change the app background color layoutRoot.setBackgroundColor(Color.RED) } // Display a negative button on alert dialog builder.setNegativeButton("No") { _, _ -> Toast.makeText(applicationContext, "You are not agree.", Toast.LENGTH_SHORT).show() } // Display a neutral button on alert dialog builder.setNeutralButton("Cancel") { _, _ -> Toast.makeText(applicationContext, "You cancelled the dialog.", Toast.LENGTH_SHORT).show() } // Finally, make the alert dialog using builder val dialog: AlertDialog = builder.create() // Display the alert dialog on app interface dialog.show() } } }
Link